Uploading to SoundCloud using PHP script without official API sign-up
SoundCloud is undoubtedly one of the most popular audio distribution platforms out there. Many podcasters and musicians use it. The current app and web uploads are good enough when it comes to distributing small amount of audio files occasionally. But wouldn't it be even better if you can create your own script that automate the uploads to SoundCloud. This is especially great, if you are building an omnichannel that can upload an audio track to multiple platforms at the same time from just a single upload.
As you might have known, SoundCloud closed its API registration for many years now. The only workable way to integrate to its backend is using IFTTT (If This Then That) service. Best of all, it is a FREE service. It also comes with an Android and iOS App.
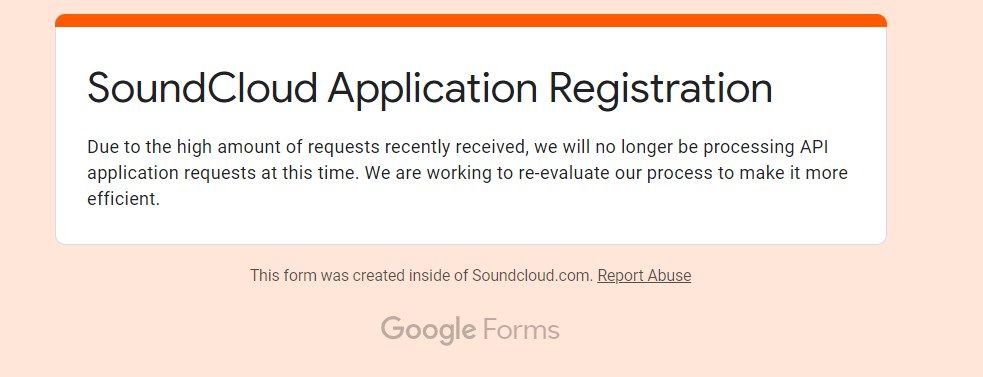
So how does this work? There could be many ways to do this. But, our method is to use RSS Feed as the trigger. IFTTT checks for any new RSS items in the provided URL. If new items have been added then it will upload to SoundCloud.
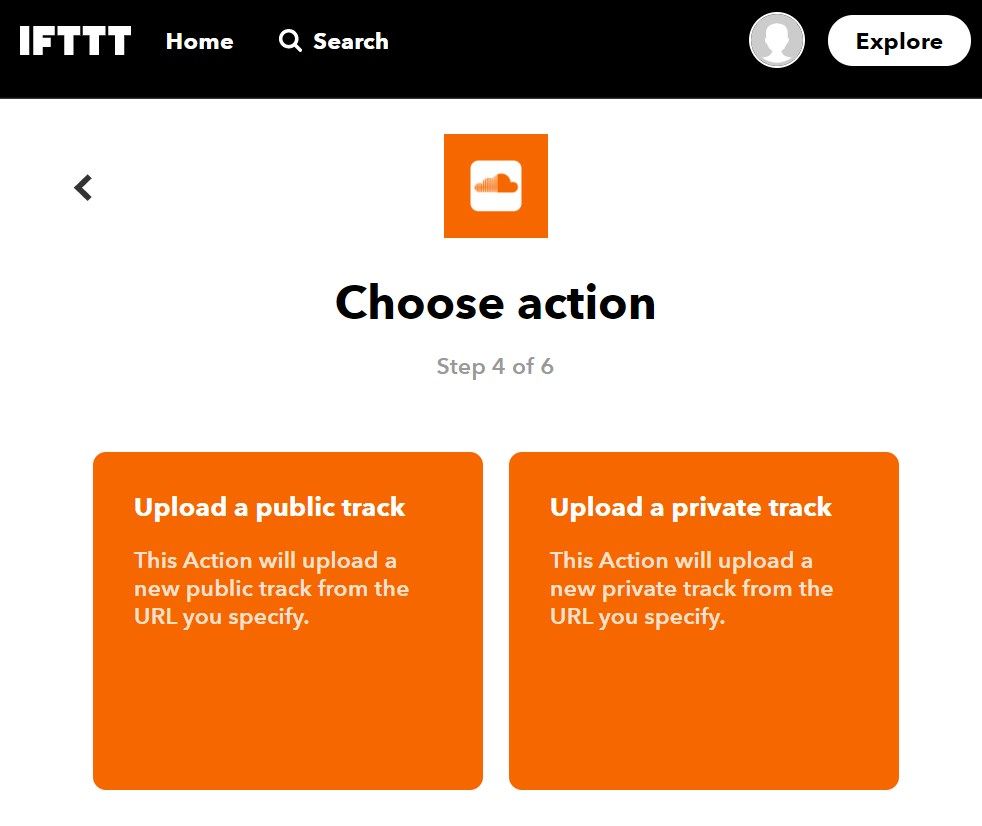
So, we need to write a simple PHP script that can generate the RSS Feed of the audio tracks we want to upload to SoundCloud. You can name this PHP file rss.php. In this example, we assume that the MP3 audio files you want to upload to SoundCloud are placed into the "temp" directory. You can modify this script to suit your needs.
<?php
//Assuming the mp3 files to be uploaded are placed into the temp folder
//Read all mp3 files inside temp folder
$tempfiles = glob("temp/*.mp3");
$rssfeed = '<?xml version="1.0" encoding="utf-8" ?>';
$rssfeed .= '<rss version="2.0">';
$rssfeed .= '<channel>';
$rssfeed .= '<title>Audio Track RSS</title>';
$rssfeed .= '<link>https://your-link-to-rss/rss.php</link>';
$rssfeed .= '<description>Audio Track</description>';
$rssfeed .= '<language>en-us</language>';
$rssfeed .= '<copyright>Your Name</copyright>';
foreach($tempfiles as $tempfile){
$title = 'Audio Track Title';
$description = '';
$link = 'https://your-link-to-mp3-folder/temp/'.basename($tempfile);
$mdate = filemtime($tempfile.'.txt');
if (!$mdate) $mdate = filemtime($tempfile);
//show if less than 1 day ago tracks, don't show older tracks
if (get_days_ago('@'.$mdate)<1) {
$rssfeed .= '<item>';
$rssfeed .= '<title>' . htmlspecialchars($title) . '</title>';
$rssfeed .= '<description>' . htmlspecialchars($description) . '</description>';
$rssfeed .= '<link>' . htmlspecialchars($link) . '</link>';
$rssfeed .= '<pubDate>' . htmlspecialchars(date("D, d M Y H:i:s O", $mdate)) . '</pubDate>';
//$rssfeed .= '<guid isPermaLink="false">' . htmlspecialchars(basename($tempfile)) . '</guid>';
$rssfeed .= '<guid>' . htmlspecialchars($link) . '</guid>';
$rssfeed .= '</item>';
$rssfeed .= "\n";
}
}
$rssfeed .= '</channel>';
$rssfeed .= '</rss>';
header('Content-Type: application/xml; charset=utf-8');
echo $rssfeed;
function get_days_ago($datetime) {
$now = new DateTime;
$ago = new DateTime($datetime);
$diff = $now->diff($ago);
$daysago = $diff->format("%a");
return $daysago;
}
?>
Now, you can sign up a free IFTTT account and head over to "Create your own applet" page. Select "New feed item" as the trigger and "SoundCloud" -> "Upload a public track" as the action.
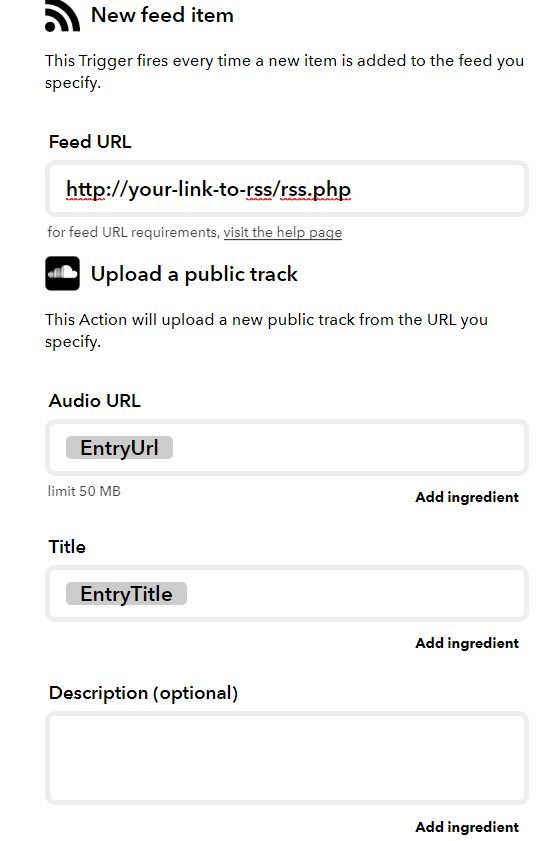
Click "Save" and you're done! You can check if the activity ran successfully under the "View Activity" page.
Do you need a custom IFTTT integration? Feel free to reach out to me to discuss your requirements.